COURSE OBJECTIVES
➢ To implement linear and non-linear data structures.
➢ To understand the different operations of search trees.
➢ To implement graph traversal algorithms and sorting algorithms.
➢ To get familiarized to binary tree traversal.
➢ To implement travelling salesman problem.
LIST OF EXPERIMENTS
1. Program to find the largest and smallest number in an unsorted array.
2. Program to implement operations on a Singly linked list.
3. Program to implement operations on a doubly linked list.
4. Program to sort the elements using insertion sort.
5. Program to sort the elements using quick sort.
6. Program to sort the elements using merge sort.
7. Program to implement a Stack using an array and Linked list.
8. Program to implement Queue using an array and Linked list.
9. Program to implement Circular Queue.
10. Program to convert an infix expression to postfix expression.
11. Program to implement BFS and DFS
12. Program to implement N Queens problem.
13. Program to implement Binary Tree Traversal
14. Program to implement Travelling Salesman Problem
COURSE OUTCOMES
On completion of the course, student will be able to
CO1: Remembering the concept of data structures through ADT including List, Stack and Queues
CO2: Understand basic concepts about stacks, queues, lists, trees and graphs
CO3: Able to apply and implement various tree traversal algorithms and ensure their correctness
CO4: Ability to analyze algorithms and develop algorithms through step by step approach in solving problems with the help of fundamental data structures.
CO5: Compare and contrast BFS and DFS.
CO6: Design applications and justify use of specific linear and binary data structures for various applications
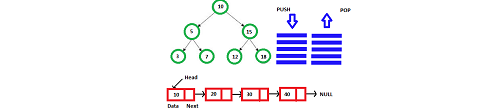
- Teacher: JESLIN SHANTHAMALAR JACOB SAMUEL
- Teacher: Roobini M S
- Teacher: Srinivasan N
- Teacher: Aishwarya R
- Teacher: Sathya Bama R
- Teacher: Prince Mary S
- Teacher: NITHYA SEKAR